浮动
div是块状元素,一个div都是独占一行,此时很多div元素在一排排列,就可以用到浮动,让竖着的盒子横着排列。
浮动属性
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>浮动</title>
<style>
/*
float 浮动
属性值:left 元素左浮动
right 元素右浮动
none 元素不浮动
浮动之后不占位置
*/
div{
width: 200px;
height: 200px;
}
.red{
background-color: red;
float: left;
}
.blue{
background-color: blue;
float: left;
/* width: 300px;
height: 300px; */
}
.green{
background-color: green;
float: left;
}
</style>
</head>
<body>
<div class="red"></div>
<div class="blue"></div>
<div class="green"></div>
</body>
</html>
多个元素浮动
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>多个元素浮动</title>
<style>
/*
float 浮动
很多个元素,一行排不下,换行往后排
*/
div{
width: 200px;
height: 200px;
}
.red{
background-color: red;
float: left;
}
.blue{
background-color: blue;
float: left;
}
.green{
background-color: green;
float: left;
}
</style>
</head>
<body>
<div class="red"></div>
<div class="blue"></div>
<div class="green"></div>
<div class="red"></div>
<div class="blue"></div>
<div class="green"></div>
<div class="red"></div>
<div class="blue"></div>
<div class="green"></div>
<div class="blue"></div>
<div class="red"></div>
<div class="blue"></div>
</body>
</html>
右浮动
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>右浮动</title>
<style>
/*
float 浮动
浮动后面会用于布局,比如一个元素在左边,一个元素在右边,就可以用浮动
左浮动:谁先浮动谁在左边
右浮动:谁先浮动谁在右边
右浮动很多个元素,一行排不下,也是换行靠右往后排
*/
div{
width: 200px;
height: 200px;
}
.red{
background-color: red;
float: right;
}
.blue{
background-color: blue;
/* float: right; */
}
.green{
background-color: green;
/* float: right; */
}
</style>
</head>
<body>
<div class="red"></div>
<div class="blue"></div>
<div class="green"></div>
</body>
</html>
特殊元素浮动
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>特殊浮动</title>
<style>
/*
float 浮动
不同大小的元素,一行不够不是另起一行,而是智能排序
能节约就节约,见缝插针的原则
*/
.red{
width: 1000px;
height: 1000px;
background-color: red;
float: left;
}
.blue{
width: 800px;
height: 800px;
background-color: blue;
float: left;
}
.green{
width: 200px;
height: 200px;
background-color: green;
float: left;
}
</style>
</head>
<body>
<div class="red"></div>
<div class="blue"></div>
<div class="green"></div>
</body>
</html>
文字
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>文字</title>
<style>
/*
float 浮动
浮动文字部分不会被挡住,文字会被挤出来
可以利用这个让文字围绕一个元素的背景周边环绕
*/
div{
width: 200px;
height: 200px;
}
.red{
background-color: red;
float: left;
}
.blue{
background-color: blue;
color: #fff;
width: 300px;
height: 300px;
}
.green{
background-color: green;
}
</style>
</head>
<body>
<div class="red"></div>
<div class="blue">你好</div>
<div class="green"></div>
</body>
</html>```
## 清除浮动
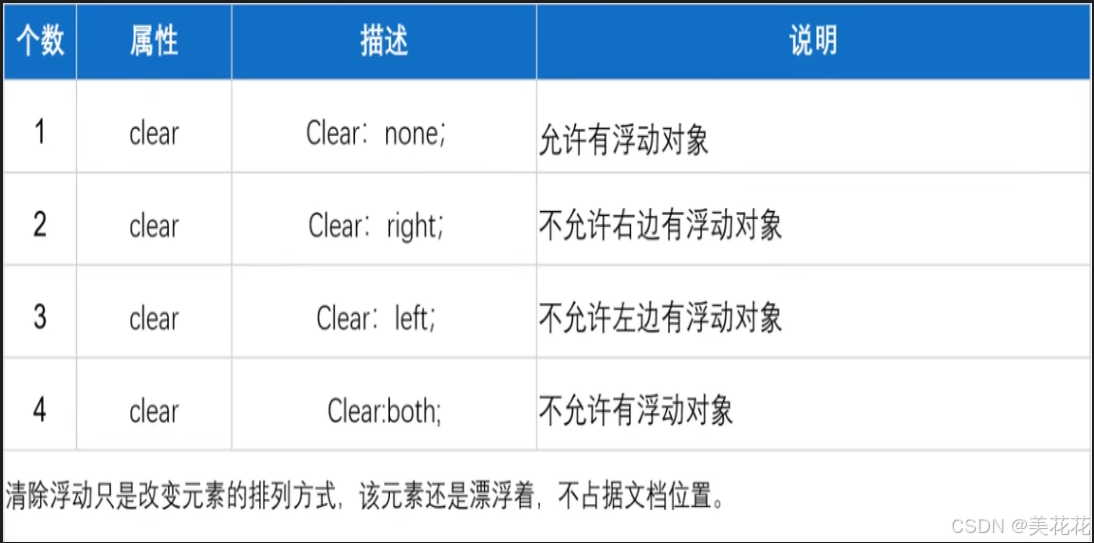
```javascript
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>清除浮动</title>
<style>
/*
高度塌陷
上面外边的盒子高度变成了0, 加了浮动之后,不占据文档位置,下面的盒子就会上来。
解决:
1. 固定高度写死
2. 清除浮动-加兄弟盒子身上,谁需要加谁身上
clear: none 允许浮动
/right 清除右浮动
/left 清除左浮动
/both 清除所有浮动
3. 给当前浮动元素后面补一个盒子,不设置宽高,加一个clear:both;属性
4. overflow: hidden;
会形成BFC,块格式化上下文,给当前元素的父盒子上加这个属性,
会自动计算内容需要占的高度,且不会影响其他元素
*/
/* 方法一:固定写死高度 */
/* .container{
height: 200px;
} */
/* 方法四:加overflow: hidden; */
.container{
overflow: hidden;
}
.box1,.box2{
width: 200px;
height: 200px;
float: left;
}
.box1{
background-color: yellow;
}
.box2{
background-color: blue;
}
.box3{
width: 300px;
height: 300px;
background-color: green;
/* 方法二:清除浮动-谁需要改变样式加谁身上 */
/* clear: left; */
}
</style>
</head>
<body>
<div class="container">
<div class="box1"></div>
<div class="box2"></div>
<!-- 方法三:给当前盒子后面补一个没有宽高的盒子,clear: left/both -->
<!-- <div style="clear: both;"></div> -->
</div>
<div class="box3"></div>
</body>
</html>
盒子模型
盒子
- CSS处理网页时,它认为每个元素都包含在一个不可见的盒子里。
- 为什么要想象成盒子呢?因为如果把所有的元素都想象成盒子,那么我们对网页的布局就相当于是摆放盒子。
- 我们只需要将相应的盒子摆放到网页中相应的位置即可完成网页的布局。
盒子模型
一个盒子我们会分成几个部分:
– 内容区(content)
– 内边距(padding)
– 边框(border)
– 外边距(margin)
内容区
- 内容区指的是盒子中放置内容的区域,也就是元素中的文本内容,子元素都是存在于内容区中的。
- 如果没有为元素设置内边距和边框,则内容区大小默认和盒子大小是一致的。
- 通过width和height两个属性可以设置内容区的大小。
- width和height属性只适用于块元素。
边框
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>盒子模型-边框</title>
<style>
.box{
/*
width-设置盒子内容区的宽度
height-设置盒子内容区的高度
width和height只是设置的盒子内容区的大小,而不是盒子整个的大小。
盒子可见框的大小是由内容区,内边距和边框共同决定的
*/
width: 100px;
height: 100px;
background-color: greenyellow;
/*
为元素设置边框-必须指定三个样式
border-width: 边框的宽度
border-color: 边框的颜色
border-style: 边框的样式
*/
/* border-width: 10px; */
/*
border-width分别指定四个边框的宽度-
如果指定了四个值,则分别设置给上右下左,顺时针顺序设置的
如果指定三个值,则这三个值分别设置给上 左右 下
如果指定两个值,则分别是上下 左右
如果指定一个值,则四边全都是该值。
除了border-width之外,CSS还给我们提供了border-xxx-width,
xxx可以是top right bottom left
专门用来设置指定边的宽度
*/
/* border-width: 10px 20px 30px 40px; */
/* border-width: 10px 20px 30px; */
/* border-width: 10px 20px; */
border-width: 10px;
/* border-color: red; */
/*
设置边框的颜色 上右下左 /上 左右 下 /上下 左右
与宽度一样,color也提供了border-xxx-color,
xxx可以是top right bottom left
专门用来设置指定边的颜色
*/
/* border-color: red yellow orange blue; */
/* border-color: red yellow orange; */
border-color: red yellow;
/*
边框的样式-border-style
可选值:none 没有边框-默认值
solid 实线
dashed 虚线
double 双线
dotted 点状边框
也可以分别指定四个边框的样式,规则与宽度和颜色一致,
同时也提供border-xxx-style来分别设置四个边的样式
*/
border-style: solid;
/* border-style: solid dashed double dotted; */
}
</style>
</head>
<body>
<div class="box"></div>
</body>
</html>
边框的其他属性
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>边框</title>
<style>
.box{
width: 200px;
height: 200px;
background-color: yellowgreen;
/*
设置边框
大部分浏览器中,边框的宽度和颜色都有默认值,而边框的默认值是none
所以如果要加边框,border-style必须写
*/
/* border-width: 10px;
border-color: red; */
/* border-style: solid; */
/*
border
边框的简写样式,
-通过它可以同时设置四个边框的样式,宽度,颜色
-没有任何的顺序要求
-border一指定就是指定四个边,不能分别指定
除了border,还有border-top,border-right,border-bottom,border-left分别设置四个边框的样式
*/
border: 10px solid red;
/* border-top: 10px solid red; */
}
.box1{
/*
如果想要给上下左都设置边框为10px,右边不需要
*/
width: 200px;
height: 200px;
background-color: yellowgreen;
/* border-top: 10px solid yellow;
border-bottom: 10px solid yellow;
border-left: 10px solid yellow; */
border: 10px solid yellow;
border-right: none;
}
</style>
</head>
<body>
<div class="box"></div>
<div class="box1"></div>
</body>
</html>
内边距
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>内边距</title>
<style>
.box{
width: 200px;
height: 200px;
background-color: yellowgreen;
border: 10px solid red;
/*
内边距 padding
指的是盒子的内容区与盒子边框之间的距离
一共有四个方向的内边距,可以通过:
padding-top --设置上内边距
padding-right --设置右内边距
padding-bottom --设置下内边距
padding-left --设置左内边距
来设置四个方向的内边距
内边距的大小会影响盒子的可见框的大小,元素的背景会延伸到内边距
盒子的大小是由内容区,内边距,和边框共同决定的
盒子的可见宽度 = border-left-width + padding-left + width + padding-right + border-right-width
盒子的可见高度 = border-top-width + padding-top + height + padding-bottom + border-bottom-width
*/
/* padding-left: 100px;
padding-top: 100px; */
/*
使用padding可以同时设置四个边框的样式,规则与border-width一致
*/
/* padding: 100px; */
/* padding: 100px 200px; */
/* padding: 100px 200px 300px; */
padding: 100px 200px 300px 400px;
}
.box1{
width: 100%;
height: 100%;
background-color: yellow;
}
</style>
</head>
<body>
<div class="box">
<div class="box1"></div>
</div>
</body>
</html>
外边距
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>内边距</title>
<style>
.box{
width: 200px;
height: 200px;
background-color: greenyellow;
border: 10px solid red;
/*
外边距 margin
指的是当前盒子与其他盒子之间的距离
他不会影响可见框的大小,而是会影响到盒子的位置
盒子由四个方向的外边距:
margin-top --设置上外边距
margin-right --设置右外边距
margin-bottom --设置下外边距
margin-left --设置左外边距
由于页面中的元素都是靠左靠上摆放的,
所以注意当我们改变上和左外边距时,会导致盒子自身的位置发生改变
而如果设置右和下边框会改变其他盒子的位置
*/
/* margin-top: 100px; */
/* margin-left: 100px; */
/* 设置右和下外边距 */
margin-bottom: 100px;
margin-right: 100px;
/*
外边距可以指定一个负值,
如果外边距设置的时负值,则元素会向反方向移动
*/
margin-top: -100px;
margin-left: -100px;
/* margin-bottom不会影响自身,会影响其他元素 */
margin-bottom: -100px;
/*
margin还可以设置为auto,auto一般只设置给水平方向的margin
如果只指定左外边距和右外边距的margin为auto,则会将会外边距设置为最大值
垂直方向外边距如果设置为auto,则外边距就是0
如果将left和right同时设置为auto,则会将两侧的外边距设置为相同的值
使元素自动在父元素中居中,我们经常将左右外边距都设置为auto
*/
margin-left: auto;
margin-right: auto;
/*
外边距可以直接简写属性margin,可以同时设置四个方向的外边距
规则与padding一样
*/
/* margin: 10px 20px 30px 40px; */
/* margin: 10px 20px 30px; */
/* margin: 10px 20px; */
margin: 10px;
/* margin: 0 auto; */
}
.box1{
width: 100px;
height: 100px;
background-color: green;
}
</style>
</head>
<body>
<div class="box"></div>
<div class="box1"></div>
</body>
</html>```
#### 垂直外边距重叠
```javascript
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>垂直外边距的重叠</title>
<style>
.box1{
width: 100px;
height: 100px;
background-color: red;
/* 设置下外边框 */
margin-bottom: 100px;
}
/*
垂直外边距的重叠(相邻,垂直两个条件)
在网页中相邻的垂直方向的外边距会发生外边距的重叠
所谓的垂直外边距的重叠是指兄弟元素之间的相邻外边距会取最大值,而不是求和
如果父子元素的垂直外边距相邻了,则子元素的外边框会设置给父亲
*/
.box2{
width: 100px;
height: 100px;
background-color: green;
/* 设置上外边框 */
margin-top: 100px;
}
.box3{
width: 200px;
height: 200px;
background-color: yellow;
/*
2.为box3设置一个上边框
3.设置一个padding-top,加上之后高度要减去1px,高度为199px
*/
/* border-top: 1px solid red; */
/* padding-top: 1px; */
/*
3.不给box4加外边距了,给box3加一个内边距 padding-top: 100px;
加上之后宽度要减去100px,height: 100px
*/
/* padding-top: 100px; */
}
.box4{
width: 100px;
height: 100px;
background-color: red;
/* 3. */
/* margin-top: 10px; */
}
</style>
</head>
<body>
<div class="box1"></div>
<!-- 可以在两个盒子之间加个元素,变成不相邻的盒子就不会发生重叠现象 -->
<!-- 111 -->
<div class="box2"></div>
<div class="box3">
<!-- 222 -->
<!-- 1. 可以加一个元素,变成不相邻的盒子 -->
<div class="box4"></div>
</div>
</body>
</html>
浏览器默认样式
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>浏览器默认样式</title>
<style>
/* body{
margin: 0;
}
p{
margin: 0;
}
ul{
margin: 0;
padding: 0;
} */
*{
margin: 0;
padding: 0;
}
div{
width: 100px;
height: 100px;
background-color: yellow;
}
</style>
</head>
<body>
<div></div>
<p>111</p>
<ul>
<li>222</li>
<li>333</li>
<li>444</li>
</ul>
</body>
</html>
内联元素的盒子模型
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>内联元素的盒模型</title>
<style>
div{
width: 100px;
height: 100px;
background-color: yellow;
}
span{
background-color: green;
}
.text1{
/* 内容区,内边距,边框,外边距 */
/*
内联元素不能设置width和height
*/
width: 100px;
height: 100px;
/*
设置水平内边距,内联元素可以设置水平方向的内边距
*/
padding-left: 100px;
padding-right: 100px;
/*
垂直方向内边距,内联元素可以设置垂直方向的内边距,但是不会影响布局(所占的位置的高度还是之前的高度)
*/
/* padding-top: 50px;
padding-bottom: 50px; */
/*
为元素设置边框,
内联元素可以设置边框,但是垂直的边框不会影响页面布局
*/
/* border: 100px solid red; */
/*
水平外边距
内联元素支持水平方向的外边距
水平外边距两个元素之间不会重叠,而是求和
*/
margin-left: 100px;
margin-right: 100px;
/*
内联外边距不支持垂直外边距
*/
margin-top: 100px;
margin-bottom: 100px;
}
.text2{
margin-left: 100px;
margin-right: 100px;
}
</style>
</head>
<body>
<span class="text1">第一个元素</span>
<span class="text2">第二个元素</span>
<span>第三个元素</span>
<span>第四个元素</span>
<div></div>
</body>
</html>
标准盒模型和怪异盒模型
box-sizing: 属性允许以特定的方式定义匹配某个区域的特定元素
/* 标准盒模型属性:content-box */
div {
box-sizing: content-box;
}
/* 怪异盒模型属性:border-box */
div {
box-sizing: border-box;
}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>标准盒模型和怪异盒模型</title>
<style>
div {
width: 50px;
height: 50px;
padding: 5px;
border: 6px solid red;
margin: 7px;
}
</style>
</head>
<body>
<div>1</div>
<div style="box-sizing: content-box">2</div>
<div style="box-sizing: border-box;">3</div>
</body>
</html>
标准盒模型和怪异盒模型的区别
标准盒模型:设置的宽度和高度只是内容content的宽高,使用box-sizing: content-box;
转化为标准盒模型。
标准盒模型下:
盒子的可见宽度 = width(content的宽度) + padding + border-width 盒子的可见高度 = height(content的高度) + padding + border-width
怪异盒模型:设置的宽度和高度是整个盒子的宽度和高度,在宽度和高度之内绘制元素的内边距和边框。使用box-sizing: border-box;转化为怪异盒模型。
怪异盒模型下:
盒子的可见宽度 = width = content + padding + border-width 盒子的可见高度 = height = content + padding + border-width
标签:color,margin,100px,笔记,height,width,day05,Learn,border From: https://blog.csdn.net/qq_58698928/article/details/143303123