Yolov8 源码解析(十一)
comments: true
description: Learn how to run inference using the Ultralytics HUB Inference API. Includes examples in Python and cURL for quick integration.
keywords: Ultralytics, HUB, Inference API, Python, cURL, REST API, YOLO, image processing, machine learning, AI integration
Ultralytics HUB Inference API
The Ultralytics HUB Inference API allows you to run inference through our REST API without the need to install and set up the Ultralytics YOLO environment locally.
Watch: Ultralytics HUB Inference API Walkthrough
Python
To access the Ultralytics HUB Inference API using Python, use the following code:
import requests
# API URL, use actual MODEL_ID
url = "https://api.ultralytics.com/v1/predict/MODEL_ID"
# Headers, use actual API_KEY
headers = {"x-api-key": "API_KEY"}
# Inference arguments (optional)
data = {"size": 640, "confidence": 0.25, "iou": 0.45}
# Load image and send request
with open("path/to/image.jpg", "rb") as image_file:
files = {"image": image_file}
response = requests.post(url, headers=headers, files=files, data=data)
print(response.json())
!!! note "Note"
Replace `MODEL_ID` with the desired model ID, `API_KEY` with your actual API key, and `path/to/image.jpg` with the path to the image you want to run inference on.
cURL
To access the Ultralytics HUB Inference API using cURL, use the following code:
curl -X POST "https://api.ultralytics.com/v1/predict/MODEL_ID" \
-H "x-api-key: API_KEY" \
-F "image=@/path/to/image.jpg" \
-F "size=640" \
-F "confidence=0.25" \
-F "iou=0.45"
!!! note "Note"
Replace `MODEL_ID` with the desired model ID, `API_KEY` with your actual API key, and `path/to/image.jpg` with the path to the image you want to run inference on.
Arguments
See the table below for a full list of available inference arguments.
Argument | Default | Type | Description |
---|---|---|---|
image |
image |
Image file to be used for inference. | |
url |
str |
URL of the image if not passing a file. | |
size |
640 |
int |
Size of the input image, valid range is 32 - 1280 pixels. |
confidence |
0.25 |
float |
Confidence threshold for predictions, valid range 0.01 - 1.0 . |
iou |
0.45 |
float |
Intersection over Union (IoU) threshold, valid range 0.0 - 0.95 . |
Response
The Ultralytics HUB Inference API returns a JSON response.
Classification
!!! Example "Classification Model"
=== "`ultralytics`"
```py
from ultralytics import YOLO
# Load model
model = YOLO("yolov8n-cls.pt")
# Run inference
results = model("image.jpg")
# Print image.jpg results in JSON format
print(results[0].tojson())
```
=== "cURL"
```py
curl -X POST "https://api.ultralytics.com/v1/predict/MODEL_ID" \
-H "x-api-key: API_KEY" \
-F "image=@/path/to/image.jpg" \
-F "size=640" \
-F "confidence=0.25" \
-F "iou=0.45"
```
=== "Python"
```py
import requests
# API URL, use actual MODEL_ID
url = "https://api.ultralytics.com/v1/predict/MODEL_ID"
# Headers, use actual API_KEY
headers = {"x-api-key": "API_KEY"}
# Inference arguments (optional)
data = {"size": 640, "confidence": 0.25, "iou": 0.45}
# Load image and send request
with open("path/to/image.jpg", "rb") as image_file:
files = {"image": image_file}
response = requests.post(url, headers=headers, files=files, data=data)
print(response.json())
```
=== "Response"
```py
{
success: true,
message: "Inference complete.",
data: [
{
class: 0,
name: "person",
confidence: 0.92
}
]
}
```
Detection
!!! Example "Detection Model"
=== "`ultralytics`"
```py
from ultralytics import YOLO
# Load model
model = YOLO("yolov8n.pt")
# Run inference
results = model("image.jpg")
# Print image.jpg results in JSON format
print(results[0].tojson())
```
=== "cURL"
```py
curl -X POST "https://api.ultralytics.com/v1/predict/MODEL_ID" \
-H "x-api-key: API_KEY" \
-F "image=@/path/to/image.jpg" \
-F "size=640" \
-F "confidence=0.25" \
-F "iou=0.45"
```
=== "Python"
```py
import requests
# API URL, use actual MODEL_ID
url = "https://api.ultralytics.com/v1/predict/MODEL_ID"
# Headers, use actual API_KEY
headers = {"x-api-key": "API_KEY"}
# Inference arguments (optional)
data = {"size": 640, "confidence": 0.25, "iou": 0.45}
# Load image and send request
with open("path/to/image.jpg", "rb") as image_file:
files = {"image": image_file}
response = requests.post(url, headers=headers, files=files, data=data)
print(response.json())
```
=== "Response"
```py
{
success: true,
message: "Inference complete.",
data: [
{
class: 0,
name: "person",
confidence: 0.92,
width: 0.4893378019332886,
height: 0.7437513470649719,
xcenter: 0.4434437155723572,
ycenter: 0.5198975801467896
}
]
}
```
OBB
!!! Example "OBB Model"
=== "`ultralytics`"
```py
from ultralytics import YOLO
# Load model
model = YOLO("yolov8n-obb.pt")
# Run inference
results = model("image.jpg")
# Print image.jpg results in JSON format
print(results[0].tojson())
```
=== "cURL"
```py
curl -X POST "https://api.ultralytics.com/v1/predict/MODEL_ID" \
-H "x-api-key: API_KEY" \
-F "image=@/path/to/image.jpg" \
-F "size=640" \
-F "confidence=0.25" \
-F "iou=0.45"
```
=== "Python"
```py
import requests
# API URL, use actual MODEL_ID
url = "https://api.ultralytics.com/v1/predict/MODEL_ID"
# Headers, use actual API_KEY
headers = {"x-api-key": "API_KEY"}
# Inference arguments (optional)
data = {"size": 640, "confidence": 0.25, "iou": 0.45}
# Load image and send request
with open("path/to/image.jpg", "rb") as image_file:
files = {"image": image_file}
response = requests.post(url, headers=headers, files=files, data=data)
print(response.json())
```
=== "Response"
```py
{
success: true,
message: "Inference complete.",
data: [
{
class: 0,
name: "person",
confidence: 0.92,
obb: [
0.669310450553894,
0.6247171759605408,
0.9847468137741089,
...
]
}
]
}
```
Segmentation
!!! Example "Segmentation Model"
=== "`ultralytics`"
```py
from ultralytics import YOLO
# Load model
model = YOLO("yolov8n-seg.pt")
# Run inference
results = model("image.jpg")
# Print image.jpg results in JSON format
print(results[0].tojson())
```
=== "cURL"
```py
curl -X POST "https://api.ultralytics.com/v1/predict/MODEL_ID" \
-H "x-api-key: API_KEY" \
-F "image=@/path/to/image.jpg" \
-F "size=640" \
-F "confidence=0.25" \
-F "iou=0.45"
```
=== "Python"
```py
import requests
# API URL, use actual MODEL_ID
url = "https://api.ultralytics.com/v1/predict/MODEL_ID"
# Headers, use actual API_KEY
headers = {"x-api-key": "API_KEY"}
# Inference arguments (optional)
data = {"size": 640, "confidence": 0.25, "iou": 0.45}
# Load image and send request
with open("path/to/image.jpg", "rb") as image_file:
files = {"image": image_file}
response = requests.post(url, headers=headers, files=files, data=data)
print(response.json())
```
=== "Response"
```py
{
success: true,
message: "Inference complete.",
data: [
{
class: 0,
name: "person",
confidence: 0.92,
segment: [0.44140625, 0.15625, 0.439453125, ...]
}
]
}
```
Pose
!!! Example "Pose Model"
=== "`ultralytics`"
```py
from ultralytics import YOLO
# Load model
model = YOLO("yolov8n-pose.pt")
# Run inference
results = model("image.jpg")
# Print image.jpg results in JSON format
print(results[0].tojson())
```
=== "cURL"
```py
curl -X POST "https://api.ultralytics.com/v1/predict/MODEL_ID" \
-H "x-api-key: API_KEY" \
-F "image=@/path/to/image.jpg" \
-F "size=640" \
-F "confidence=0.25" \
-F "iou=0.45"
```
=== "Python"
```py
import requests
# API URL, use actual MODEL_ID
url = "https://api.ultralytics.com/v1/predict/MODEL_ID"
# Headers, use actual API_KEY
headers = {"x-api-key": "API_KEY"}
# Inference arguments (optional)
data = {"size": 640, "confidence": 0.25, "iou": 0.45}
# Load image and send request
with open("path/to/image.jpg", "rb") as image_file:
files = {"image": image_file}
response = requests.post(url, headers=headers, files=files, data=data)
print(response.json())
```
=== "Response"
```py
{
success: true,
message: "Inference complete.",
data: [
{
class: 0,
name: "person",
confidence: 0.92,
keypoints: [
0.5290805697441101,
0.20698919892311096,
1.0,
0.5263055562973022,
0.19584226608276367,
1.0,
0.5094948410987854,
0.19120082259178162,
1.0,
...
]
}
]
}
```
comments: true
description: Explore seamless integrations between Ultralytics HUB and platforms like Roboflow. Learn how to import datasets, train models, and more.
keywords: Ultralytics HUB, Roboflow integration, dataset import, model training, AI, machine learning
Ultralytics HUB Integrations
Learn about Ultralytics HUB integrations with various platforms and formats.
Datasets
Seamlessly import your datasets in Ultralytics HUB for model training.
After a dataset is imported in Ultralytics HUB, you can train a model on your dataset just like you would using the Ultralytics HUB datasets.
Roboflow
You can easily filter the Roboflow datasets on the Ultralytics HUB Datasets page.
Ultralytics HUB supports two types of integrations with Roboflow, Universe and Workspace.
Universe
The Roboflow Universe integration allows you to import one dataset at a time into Ultralytics HUB from Roboflow.
Import
When you export a Roboflow dataset, select the Ultralytics HUB format. This action will redirect you to Ultralytics HUB and trigger the Dataset Import dialog.
You can import your Roboflow dataset by clicking on the Import button.
Next, train a model on your dataset.
Remove
Navigate to the Dataset page of the Roboflow dataset you want to remove, open the dataset actions dropdown and click on the Remove option.
??? tip "Tip"
You can remove an imported [Roboflow](https://roboflow.com/?ref=ultralytics) dataset directly from the [Datasets](https://hub.ultralytics.com/datasets) page.
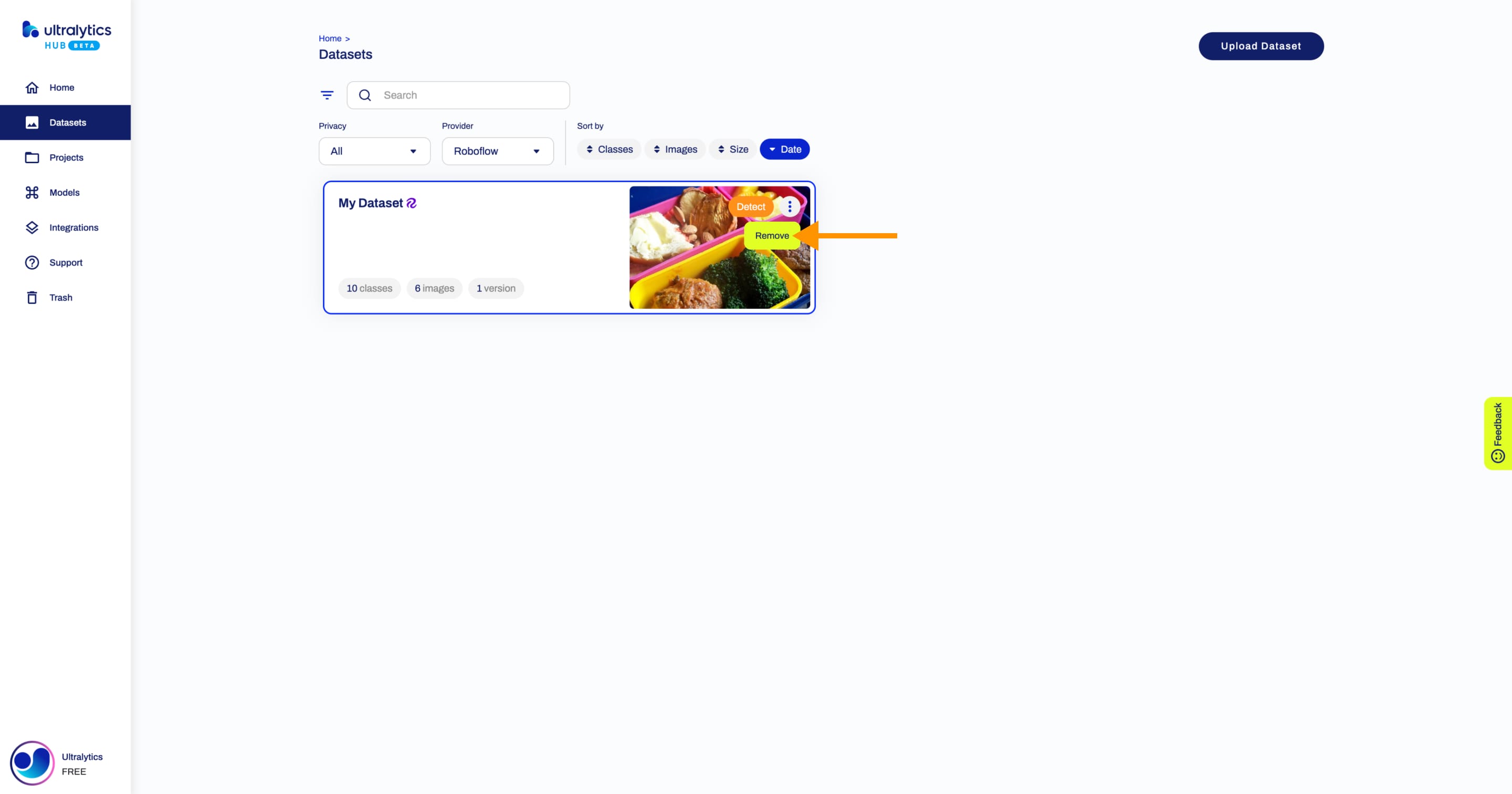
Workspace
The Roboflow Workspace integration allows you to import an entire Roboflow Workspace at once into Ultralytics HUB.
Import
Navigate to the Integrations page by clicking on the Integrations button in the sidebar.
Type your Roboflow Workspace private API key and click on the Add button.
??? tip "Tip"
You can click on the **Get my API key** button which will redirect you to the settings of your [Roboflow](https://roboflow.com/?ref=ultralytics) Workspace from where you can obtain your private API key.
This will connect your Ultralytics HUB account with your Roboflow Workspace and make your Roboflow datasets available in Ultralytics HUB.
Next, train a model on your dataset.
Remove
Navigate to the Integrations page by clicking on the Integrations button in the sidebar and click on the Unlink button of the Roboflow Workspace you want to remove.
??? tip "Tip"
You can remove a connected [Roboflow](https://roboflow.com/?ref=ultralytics) Workspace directly from the Dataset page of one of the datasets from your [Roboflow](https://roboflow.com/?ref=ultralytics) Workspace.
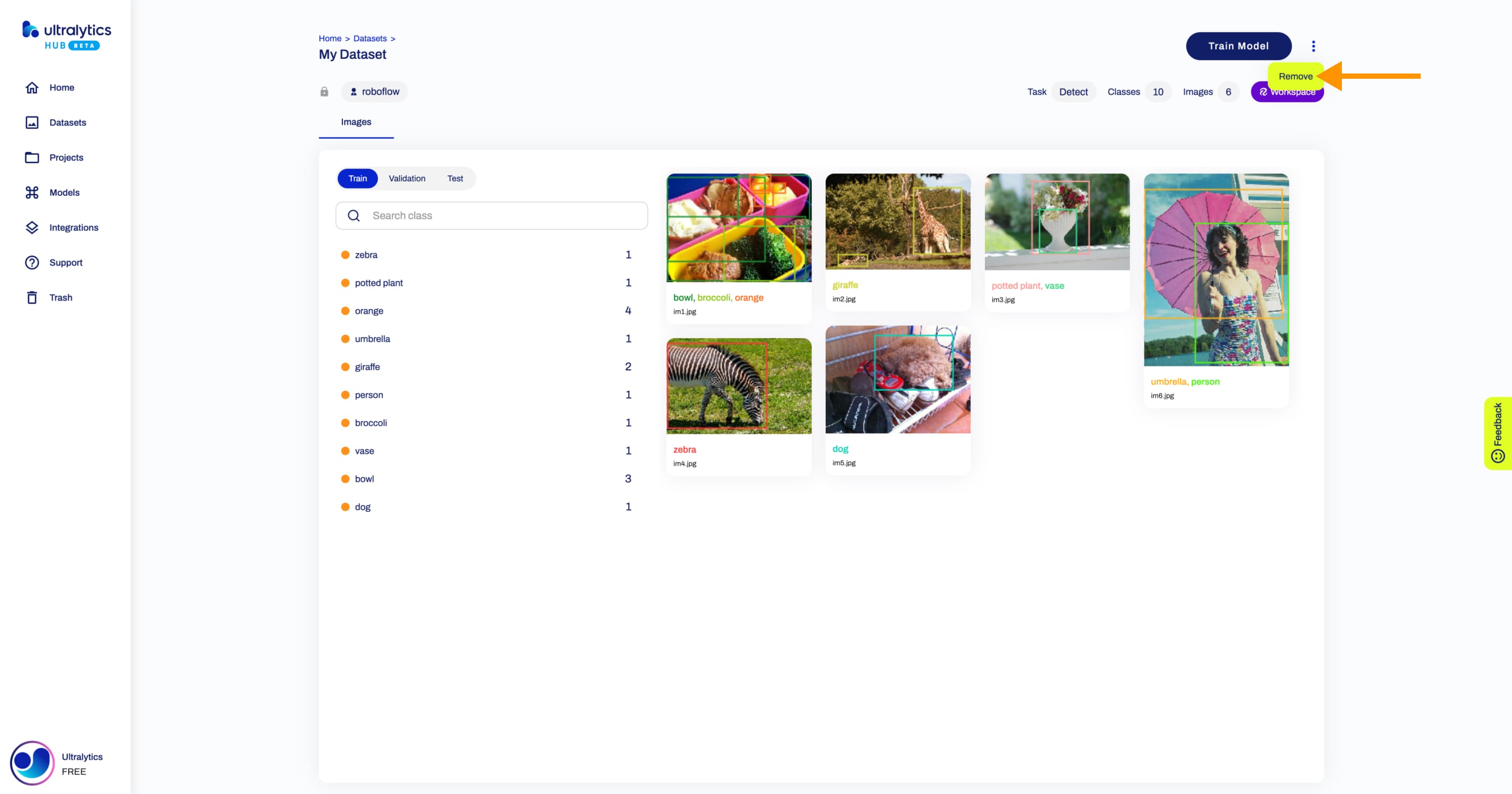
??? tip "Tip"
You can remove a connected [Roboflow](https://roboflow.com/?ref=ultralytics) Workspace directly from the [Datasets](https://hub.ultralytics.com/datasets) page.
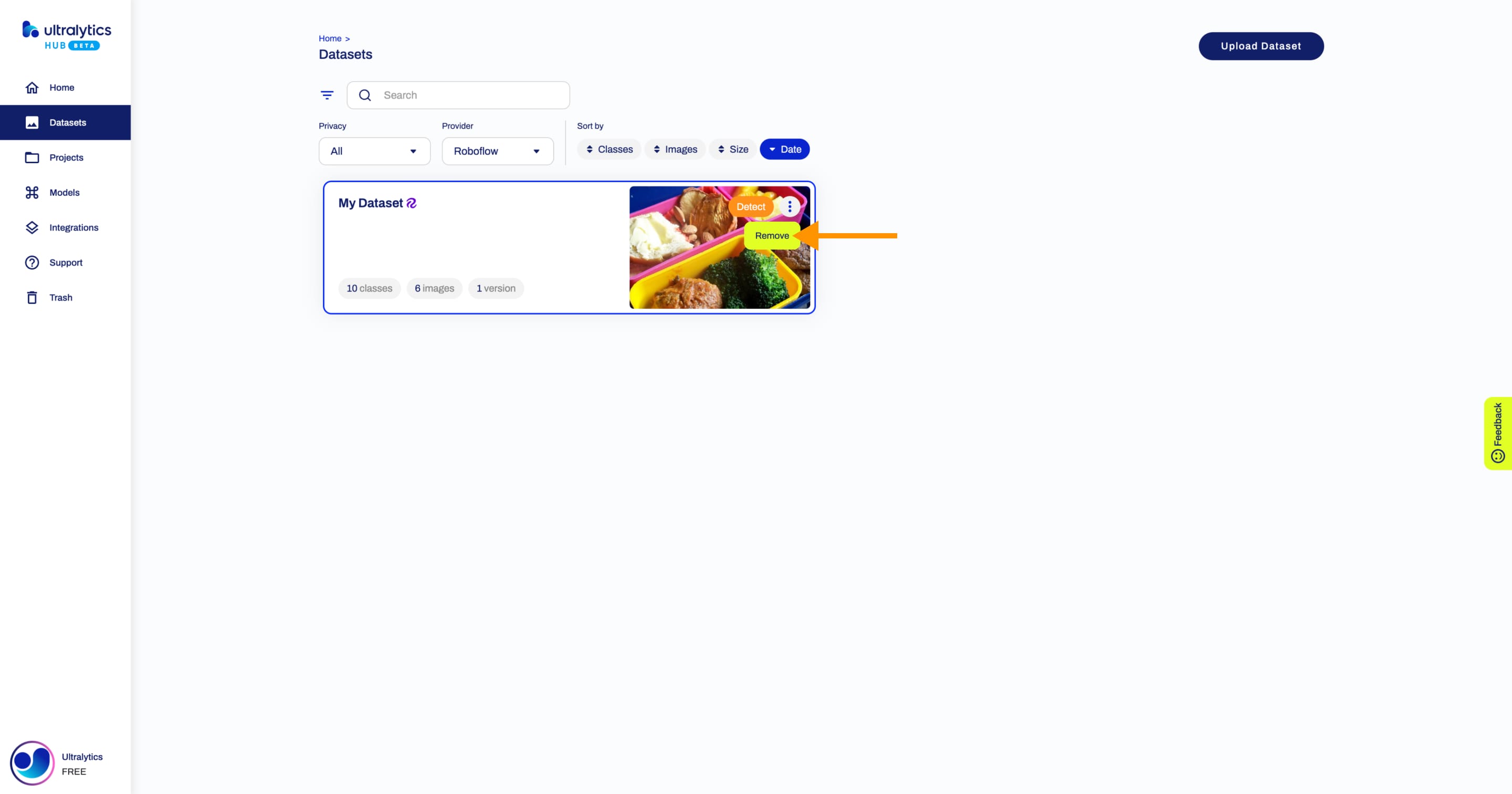
Models
Exports
After you train a model, you can export it to 13 different formats, including ONNX, OpenVINO, CoreML, TensorFlow, Paddle and many others.
The available export formats are presented in the table below.
Format | format Argument |
Model | Metadata | Arguments |
---|---|---|---|---|
PyTorch | - | yolov8n.pt |
✅ | - |
TorchScript | torchscript |
yolov8n.torchscript |
✅ | imgsz , optimize , batch |
ONNX | onnx |
yolov8n.onnx |
✅ | imgsz , half , dynamic , simplify , opset , batch |
OpenVINO | openvino |
yolov8n_openvino_model/ |
✅ | imgsz , half , int8 , batch |
TensorRT | engine |
yolov8n.engine |
✅ | imgsz , half , dynamic , simplify , workspace , int8 , batch |
CoreML | coreml |
yolov8n.mlpackage |
✅ | imgsz , half , int8 , nms , batch |
TF SavedModel | saved_model |
yolov8n_saved_model/ |
✅ | imgsz , keras , int8 , batch |
TF GraphDef | pb |
yolov8n.pb |
❌ | imgsz , batch |
TF Lite | tflite |
yolov8n.tflite |
✅ | imgsz , half , int8 , batch |
TF Edge TPU | edgetpu |
yolov8n_edgetpu.tflite |
✅ | imgsz , batch |
TF.js | tfjs |
yolov8n_web_model/ |
✅ | imgsz , half , int8 , batch |
PaddlePaddle | paddle |
yolov8n_paddle_model/ |
✅ | imgsz , batch |
NCNN | ncnn |
yolov8n_ncnn_model/ |
✅ | imgsz , half , batch |