10个复杂的C# 代码片段
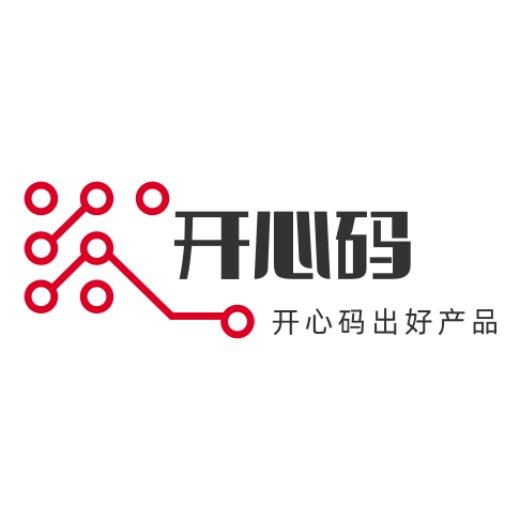

作为一名使用C#的开发人员,你经常会遇到需要复杂的代码解决方案的情况。在本文中,我们将探讨10个代码片段,用C#解决各种复杂情况。这些片段旨在帮助你解决具有挑战性的问题,并扩展你对这门编程语言的理解。
1、使用任务并行性进行多线程编程
多线程可以大大提升应用程序的性能。通过使用System.Threading.Tasks命名空间中的Task类,你可以实现高效的并行执行。以下是使用任务进行并行处理的示例:
using System;
using System.Threading.Tasks;
class Program
{
static async Task Main(string[] args)
{
Task<int> task1 = Task.Run(() => CalculateSomeValue());
Task<int> task2 = Task.Run(() => CalculateAnotherValue());
await Task.WhenAll(task1, task2);
int result1 = task1.Result;
int result2 = task2.Result;
Console.WriteLine($"Result 1: {result1}, Result 2: {result2}");
}
static int CalculateSomeValue() { /* Implementation */ }
static int CalculateAnotherValue() { /* Implementation */ }
}
2、异步文件I/O
处理异步文件操作对于响应性应用程序至关重要。async 和 await 关键字简化了异步编程。以下是如何进行异步文件读取的示例:
using System;
using System.IO;
using System.Threading.Tasks;
class Program
{
static async Task Main(string[] args)
{
string filePath = "data.txt";
using (StreamReader reader = new StreamReader(filePath))
{
string content = await reader.ReadToEndAsync();
Console.WriteLine(content);
}
}
}
3、LINQ Join and Group By
LINQ提供了强大的查询功能。结合join和group by操作可以帮助你高效地操作数据。考虑以下示例,用于从两个集合中连接和分组数据:
using System;
using System.Collections.Generic;
using System.Linq;
class Program
{
static void Main(string[] args)
{
List<Order> orders = GetOrders();
List<Product> products = GetProducts();
var query = from order in orders
join product in products on order.ProductId equals product.Id
group order by product.Category into categoryGroup
select new
{
Category = categoryGroup.Key,
TotalAmount = categoryGroup.Sum(o => o.Amount)
};
foreach (var category in query)
{
Console.WriteLine($"Category: {category.Category}, Total Amount: {category.TotalAmount}");
}
}
// Define Order and Product classes here
}
4、Complex Regular Expressions
正则表达式为模式匹配和文本处理提供了多功能工具。在这个示例中,我们使用复杂的正则表达式模式来从字符串中提取URL:
using System;
using System.Text.RegularExpressions;
class Program
{
static void Main(string[] args)
{
string input = "Visit my website at https://example.com and learn more!";
string pattern = @"https?://\S+";
MatchCollection matches = Regex.Matches(input, pattern);
foreach (Match match in matches)
{
Console.WriteLine(match.Value);
}
}
}
5、http://ASP.NET Core 中的依赖注入
在现代软件架构中,依赖注入对于构建模块化和可测试的应用程序至关重要。http://ASP.NET Core提供了一个强大的依赖注入框架。以下是演示如何在http://ASP.NET Core应用程序中配置和使用依赖注入的代码片段:
using System;
using Microsoft.Extensions.DependencyInjection;
class Program
{
static void Main(string[] args)
{
var serviceProvider = new ServiceCollection()
.AddTransient<IMessageService, EmailService>()
.BuildServiceProvider();
var messageService = serviceProvider.GetService<IMessageService>();
messageService.SendMessage("Hello, Dependency Injection!");
// 定义IMessageService和EmailService接口/类
}
}
6、自定义异常处理
创建自定义异常允许你更有效地处理特定的错误情况。以下是定义和使用自定义异常的示例:
using System;
class CustomException : Exception
{
public CustomException(string message) : base(message) { }
}
class Program
{
static void Main(string[] args)
{
try
{
int result = PerformComplexOperation();
if (result < 0)
{
throw new CustomException("Negative result is not allowed.");
}
}
catch (CustomException ex)
{
Console.WriteLine($"Custom Exception: {ex.Message}");
}
}
static int PerformComplexOperation() { /* 实现 */ }
}
7、委托和事件
委托和事件对于事件驱动编程至关重要。在这个示例中,我们演示了如何使用自定义委托和事件进行组件间的通信:
using System;
class Program
{
delegate void EventHandler(string message);
class Publisher
{
public event EventHandler RaiseEvent;
public void DoSomething()
{
Console.WriteLine("Something is happening...");
RaiseEvent?.Invoke("Event raised from Publisher.");
}
}
class Subscriber
{
public Subscriber(Publisher publisher)
{
publisher.RaiseEvent += HandleEvent;
}
void HandleEvent(string message)
{
Console.WriteLine($"Event handled: {message}");
}
}
static void Main(string[] args)
{
Publisher publisher = new Publisher();
Subscriber subscriber = new Subscriber(publisher);
publisher.DoSomething();
}
}
8、Entity Framework 复杂查询
Entity Framework简化了数据库交互。使用LINQ可以实现涉及多个表的复杂查询。以下是查询相关数据的示例:
using System;
using System.Linq;
class Program
{
static void Main(string[] args)
{
using (var dbContext = new ApplicationDbContext())
{
var query = from order in dbContext.Orders
join customer in dbContext.Customers on order.CustomerId equals customer.Id
where order.Amount > 1000
select new
{
CustomerName = customer.Name,
OrderAmount = order.Amount
};
foreach (var result in query)
{
Console.WriteLine($"Customer: {result.CustomerName}, Amount: {result.OrderAmount}");
}
}
}
}
customer.idusing System;
using System.Linq;
class Program
{
static void Main(string[] args)
{
using (var dbContext = new ApplicationDbContext())
{
var query = from order in dbContext.Orders
join customer in dbContext.Customers on order.CustomerId equals customer.Id
where order.Amount > 1000
select new
{
CustomerName = customer.Name,
OrderAmount = order.Amount
};
foreach (var result in query)
{
Console.WriteLine($"Customer: {result.CustomerName}, Amount: {result.OrderAmount}");
}
}
}
}
9、垃圾回收和IDisposable
管理资源和内存对于高效的应用程序至关重要。实现IDisposable模式允许你控制非托管资源的处理。以下是一个实现IDisposable的类的示例:
using System;
class Resource : IDisposable
{
private bool _disposed = false;
public void Dispose()
{
Dispose(true);
GC.SuppressFinalize(this);
}
protected virtual void Dispose(bool disposing)
{
if (!_disposed)
{
if (disposing)
{
// 处理托管资源
}
// 处理非托管资源
_disposed = true;
}
}
~Resource()
{
Dispose(false);
}
}
class Program
{
static void Main(string[] args)
{
using (var resource = new Resource())
{
// 使用资源
}
}
}
10、高级反射
反射允许你动态检查和操作程序集、类型和对象。以下是使用反射获取类中方法信息的示例:
using System;
using System.Reflection;
class Program
{
static void Main(string[] args)
{
Type type = typeof(MyClass);
MethodInfo[] methods = type.GetMethods();
foreach (MethodInfo method in methods)
{
Console.WriteLine($"Method: {method.Name}");
ParameterInfo[] parameters = method.GetParameters();
foreach (ParameterInfo parameter in parameters)
{
Console.WriteLine($"Parameter: {parameter.Name}, Type: {parameter.ParameterType}");
}
}
}
}
class MyClass
{
public void Method1(int value) { /* 实现 */ }
public void Method2(string text) { /* 实现 */ }
}
这10个复杂的C#代码片段涵盖了多种情景,从多线程到高级反射。通过深入研究这些示例,你将更深入地了解C#的多功能性以及其处理复杂编程挑战的能力。
如果喜欢我的文章,可以收藏它,并且关注我,我每天给您带来开发技巧,◾w◾x ➡ rjf1979编辑于 2023-10-31 12:02・IP 属地上海 标签:10,片段,string,C#,void,System,static,using,class From: https://www.cnblogs.com/sexintercourse/p/17912390.html