数据统计(图形报表)
Apache ECharts
- Apache ECharts是一款基于Javascript的数据可视化图表库,提供直观,生动,可交互,可个性化定制的数据可视化图表。
- 官网地址:https://echarts.apache.org/zh/index.html。
- 使用Echarts,重点在于研究当前图表所需的数据格式。通常是需要后端提供符合格式要求的动态数据,然后响应给前端来展示图表。
营业额统计
需求分析和设计
产品原型
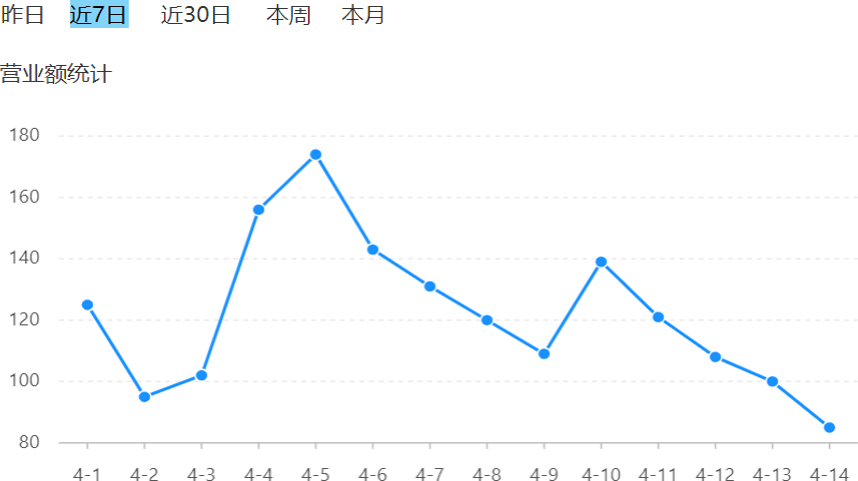
业务功能
- 营业额指订单状态为已完成的订单金额合计。
- 基于可视化报表的折线图展示营业额数据,X轴为日期,Y轴为营业额。
- 根据时间选择区间,展示每天的营业额数据。
接口设计
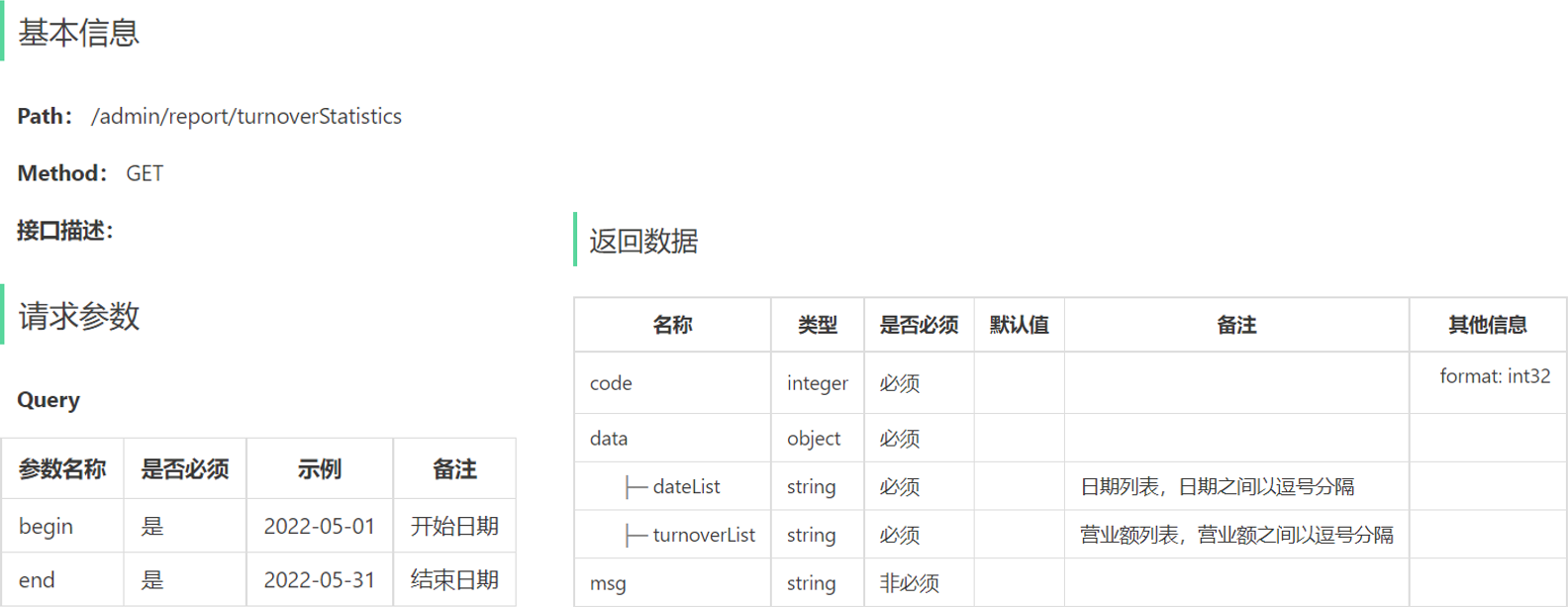
代码开发
- 根据接口定义设计对应的VO:
@Data
@Builder
@NoArgsConstructor
@AllArgsConstructor
public class TurnoverReportVO implements Serializable {
//日期,以逗号分隔,例如:2022-10-01,2022-10-02,2022-10-03
private String dateList;
//营业额,以逗号分隔,例如:406.0,1520.0,75.0
private String turnoverList;
}
- 根据接口定义创建ReportController,并在其中创建turnoverStatistics方法:
@RequestMapping("/admin/report")
@RestController
@Slf4j
@Api(tags = "数据统计相关接口")
public class ReportController {
@Autowired
private ReportService reportService;
@GetMapping("/turnoverStatistics")
@ApiOperation("营业额统计")
public Result<TurnoverReportVO> turnoverStatistics(
@DateTimeFormat(pattern = "yyyy-MM-dd") LocalDate begin,
@DateTimeFormat(pattern = "yyyy-MM-dd") LocalDate end) {
log.info("营业额统计:{}~{}", begin, end);
TurnoverReportVO turnoverReportVO = reportService.getTurnoverStatistics(begin, end);
return Result.success(turnoverReportVO);
}
}
- 创建ReportService接口,声明getTurnoverStatistics方法:
public interface ReportService {
TurnoverReportVO getTurnoverStatistics(LocalDate begin, LocalDate end);
}
- 创建ReportServiceImpl实现类,实现getTurnoverStatistics方法:
@Service
@Slf4j
public class ReportServiceImpl implements ReportService {
@Autowired
private OrderMapper orderMapper;
@Override
public TurnoverReportVO getTurnoverStatistics(LocalDate begin, LocalDate end) {
List<LocalDate> dateList = new ArrayList<>(); //当前集合用于存放从begin到end范围内每天的日期
List<Double> turnoverList = new ArrayList<>(); //当前集合用于存放从begin到end范围内每天最早和最晚的时间
dateList.add(begin);
//将范围内的日期存到集合中
while (!begin.equals(end)) {
begin = begin.plusDays(1); //日期加一
dateList.add(begin);
}
for (LocalDate date : dateList) {
LocalDateTime beginTime = LocalDateTime.of(date, LocalTime.MIN); //当天最早的时间
LocalDateTime endTime = LocalDateTime.of(date, LocalTime.MAX); //当天最晚的时间
//查询date对应日期的营业额,即状态为已完成的订单的总金额
Map map = new HashMap();
map.put("begin", beginTime);
map.put("end", endTime);
map.put("status", Orders.COMPLETED);
Double turnover = orderMapper.sumByMap(map); //查询当天的营业额
turnover = turnover == null ? 0.0 : turnover; //防止查询结果为null
turnoverList.add(turnover);
}
//将集合转换为字符串
String dateListString = StringUtils.join(dateList, ",");
String turnoverListString = StringUtils.join(turnoverList, ",");
//构造TurnoverReportVO并返回
TurnoverReportVO turnoverReportVO = TurnoverReportVO.builder()
.dateList(dateListString)
.turnoverList(turnoverListString)
.build();
return turnoverReportVO;
}
}
- 在OrderMapper接口声明sumByMap方法:
Double sumByMap(Map map);
- 在OrderMapper.xml文件中编写动态SQL:
<select id="sumByMap" resultType="java.lang.Double">
select sum(amount) from orders
<where>
<if test="begin != null">
and order_time > #{begin}
</if>
<if test="end != null">
and order_time < #{end}
</if>
<if test="status != null">
and status = #{status}
</if>
</where>
</select>
功能测试
可以通过如下方式进行测试:接口文档测试,前后端联调测试。
用户统计
需求分析和设计
产品原型
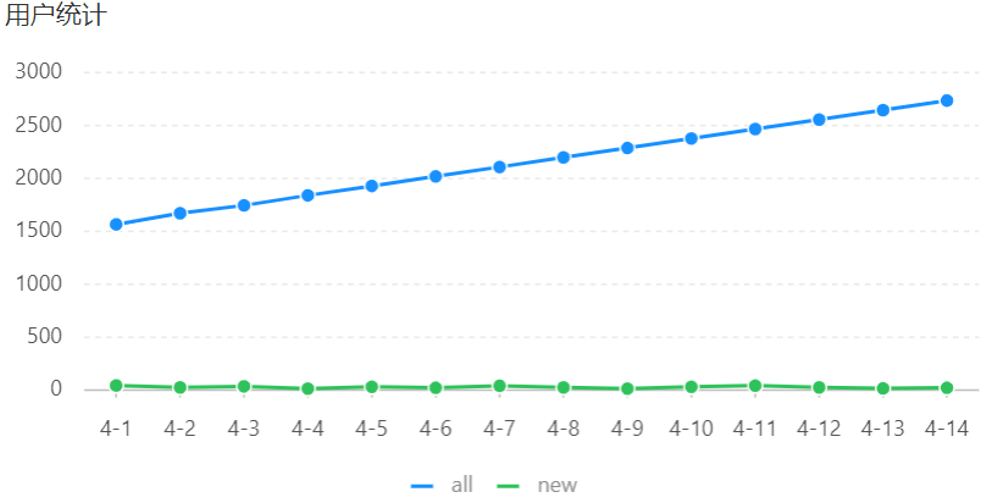
业务规则
- 基于可视化报表的折线图展示用户数据,X轴为日期,Y轴为用户数。
- 根据时间选择区间,展示每天的用户总量和新增用户量数据。
接口设计
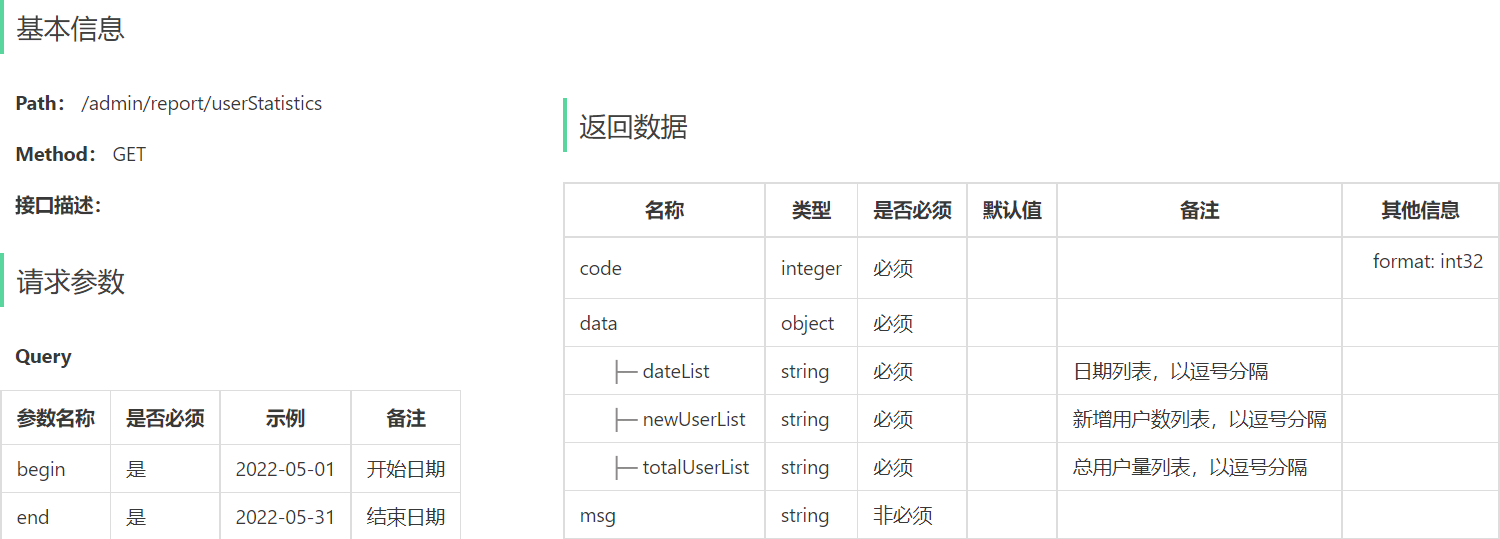
代码开发
- 根据用户统计接口的返回结果设计VO:
@Data
@Builder
@NoArgsConstructor
@AllArgsConstructor
public class UserReportVO implements Serializable {
//日期,以逗号分隔,例如:2022-10-01,2022-10-02,2022-10-03
private String dateList;
//用户总量,以逗号分隔,例如:200,210,220
private String totalUserList;
//新增用户,以逗号分隔,例如:20,21,10
private String newUserList;
}
- 根据接口定义,在ReportController中创建userStatistics方法:
@GetMapping("/userStatistics")
@ApiOperation("用户统计")
public Result<UserReportVO> userStatistics(
@DateTimeFormat(pattern = "yyyy-MM-dd") LocalDate begin,
@DateTimeFormat(pattern = "yyyy-MM-dd") LocalDate end) {
log.info("用户统计:{}~{}", begin, end);
UserReportVO userReportVO = reportService.getUserStatistics(begin, end);
return Result.success(userReportVO);
}
- 在ReportService接口中声明getUserStatistics方法:
UserReportVO getUserStatistics(LocalDate begin, LocalDate end);
- 在ReportServiceImpl实现类中实现getUserStatistics方法:
@Autowired
private UserMapper userMapper;
@Override
public UserReportVO getUserStatistics(LocalDate begin, LocalDate end) {
List<LocalDate> dateList = new ArrayList<>(); //当前集合用于存放从begin到end范围内每天的日期
List<Integer> totalUserList = new ArrayList<>(); //当前集合用于存放从begin到end范围内每天的总用户数
List<Integer> newUserList = new ArrayList<>(); //当前集合用于存放从begin到end范围内每天新增的用户数
dateList.add(begin);
//将范围内的容器存到集合中
while (!begin.equals(end)) {
begin = begin.plusDays(1); //日期加一
dateList.add(begin);
}
for (LocalDate date : dateList) {
LocalDateTime beginTime = LocalDateTime.of(date, LocalTime.MIN); //当天最早的时间
LocalDateTime endTime = LocalDateTime.of(date, LocalTime.MAX); //当天最晚的时间
//查询date对应日期的总人数和当天新增的用户数
Map map = new HashMap();
map.put("end", endTime);
Integer totalUser = userMapper.countByMap(map); //总用户数量
map.put("begin", beginTime);
Integer newUser = userMapper.countByMap(map); //新增用户数量
totalUserList.add(totalUser);
newUserList.add(newUser);
}
//将集合转换为字符串
String dateListString = StringUtils.join(dateList, ",");
String totalUserListString = StringUtils.join(totalUserList, ",");
String newUserListString = StringUtils.join(newUserList, ",");
//构造UserReportVO并返回
UserReportVO userReportVO = UserReportVO.builder()
.dateList(dateListString)
.totalUserList(totalUserListString)
.newUserList(newUserListString)
.build();
return userReportVO;
}
- 在UserMapper接口中声明countByMap方法:
Integer countByMap(Map map);
- 在UserMapper.xml文件中编写动态SQL:
<select id="countByMap" resultType="java.lang.Integer">
select count(*) from user
<where>
<if test="begin != null">
and create_time > #{begin}
</if>
<if test="end != null">
and create_time < #{end}
</if>
</where>
</select>
功能测试
可以通过如下方式进行测试:接口文档测试,前后端联调测试。
订单统计
需求分析和设计
产品原型
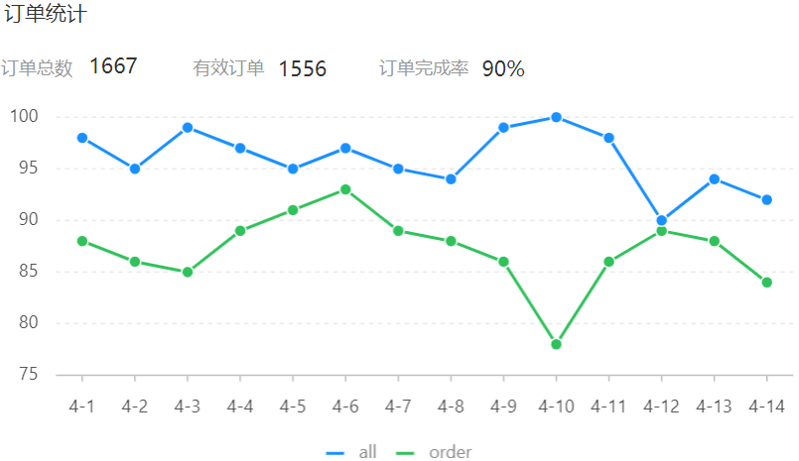
业务功能
- 有效订单指状态为 “已完成” 的订单。
- 基于可视化报表的折线图展示订单数据,X轴为日期,Y轴为订单数量。
- 根据时间选择区间,展示每天的订单总数和有效订单数。
- 展示所选时间区间内的有效订单数、总订单数、订单完成率,订单完成率 = 有效订单数 / 总订单数 * 100%。
接口设计
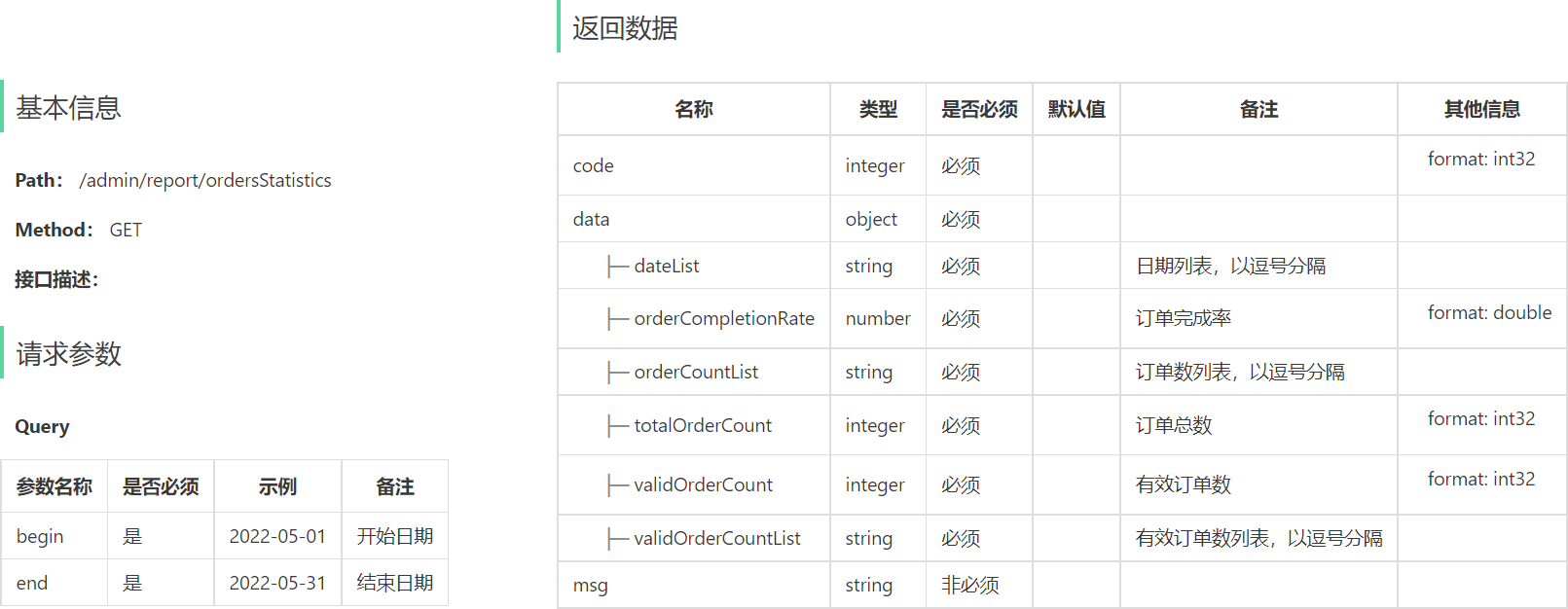
代码开发
- 根据订单统计接口的返回结果设计VO:
@Data
@Builder
@NoArgsConstructor
@AllArgsConstructor
public class OrderReportVO implements Serializable {
//日期,以逗号分隔,例如:2022-10-01,2022-10-02,2022-10-03
private String dateList;
//每日订单数,以逗号分隔,例如:260,210,215
private String orderCountList;
//每日有效订单数,以逗号分隔,例如:20,21,10
private String validOrderCountList;
//订单总数
private Integer totalOrderCount;
//有效订单数
private Integer validOrderCount;
//订单完成率
private Double orderCompletionRate;
}
- 在ReportController中根据订单统计接口创建ordersStatistics方法:
@GetMapping("/ordersStatistics")
@ApiOperation("订单统计")
public Result<OrderReportVO> ordersStatistics(
@DateTimeFormat(pattern = "yyyy-MM-dd") LocalDate begin,
@DateTimeFormat(pattern = "yyyy-MM-dd") LocalDate end) {
log.info("订单统计:{}~{}", begin, end);
OrderReportVO orderReportVO = reportService.getOrdersStatistics(begin, end);
return Result.success(orderReportVO);
}
- 在ReportService接口中声明getOrdersStatistics方法:
OrderReportVO getOrdersStatistics(LocalDate begin, LocalDate end);
- 在ReportServiceImpl实现类中实现getOrdersStatistics方法:
@Override
public OrderReportVO getOrdersStatistics(LocalDate begin, LocalDate end) {
List<LocalDate> dateList = getDateList(begin, end); //当前集合用于存放从begin到end范围内每天的日期
List<Integer> orderCountList = new ArrayList<>(); //当前集合用于存放从begin到end范围内每天的订单数
List<Integer> validOrderCountList = new ArrayList<>(); //当前集合用于存放从begin到end范围内每天的有效订单数
for (LocalDate date : dateList) {
LocalDateTime beginTime = LocalDateTime.of(date, LocalTime.MIN); //当天最早的时间
LocalDateTime endTime = LocalDateTime.of(date, LocalTime.MAX); //当天最晚的时间
//查询date对应日期的订单数和有效订单数
Map map = new HashMap();
map.put("begin", beginTime);
map.put("end", endTime);
Integer orderCount = orderMapper.countByMap(map); //查询订单数
map.put("status", Orders.COMPLETED);
Integer validOrderCount = orderMapper.countByMap(map); //查询有效订单数
orderCountList.add(orderCount);
validOrderCountList.add(validOrderCount);
}
Integer totalOrderCount = orderCountList.stream().reduce(Integer::sum).get(); //计算订单总数
Integer validOrderCount = validOrderCountList.stream().reduce(Integer::sum).get(); //计算有效订单总数
Double orderCompletionRate = totalOrderCount == 0 ? 0.0 : validOrderCount.doubleValue() / totalOrderCount; //计算订单完成率
//将集合转换为字符串
String dateListString = StringUtils.join(dateList, ",");
String orderCountListString = StringUtils.join(orderCountList, ",");
String validOrderCountListString = StringUtils.join(validOrderCountList, ",");
//构造OrderReportVO并返回
OrderReportVO orderReportVO = OrderReportVO.builder()
.dateList(dateListString)
.orderCountList(orderCountListString)
.validOrderCountList(validOrderCountListString)
.totalOrderCount(totalOrderCount)
.validOrderCount(validOrderCount)
.orderCompletionRate(orderCompletionRate)
.build();
return orderReportVO;
}
- 在ReportServiceImpl实现类中提供私有方法getDateList:
private List<LocalDate> getDateList(LocalDate begin, LocalDate end) {
List<LocalDate> dateList = new ArrayList<>(); //当前集合用于存放从begin到end范围内每天的日期
//将范围内的日期存到集合中
dateList.add(begin);
while (!begin.equals(end)) {
begin = begin.plusDays(1); //日期加一
dateList.add(begin);
}
return dateList;
}
- 改造ReportServiceImpl实现类中的getTurnoverStatistics方法和getUserStatistics方法:
public TurnoverReportVO getTurnoverStatistics(LocalDate begin, LocalDate end) {
List<LocalDate> dateList = getDateList(begin, end); //当前集合用于存放从begin到end范围内每天的日期
...
}
public UserReportVO getUserStatistics(LocalDate begin, LocalDate end) {
List<LocalDate> dateList = getDateList(begin,end); //当前集合用于存放从begin到end范围内每天的日期
...
}
- 在OrderMapper接口中声明countByMap方法:
Integer countByMap(Map map);
- 在OrderMapper.xml文件中编写动态SQL:
<select id="countByMap" resultType="java.lang.Integer">
select count(*) from orders
<where>
<if test="begin != null">
and order_time > #{begin}
</if>
<if test="end != null">
and order_time < #{end}
</if>
<if test="status != null">
and status = #{status}
</if>
</where>
</select>
功能测试
可以通过如下方式进行测试:接口文档测试,前后端联调。
销量排名Top10
需求分析和设计
产品原型
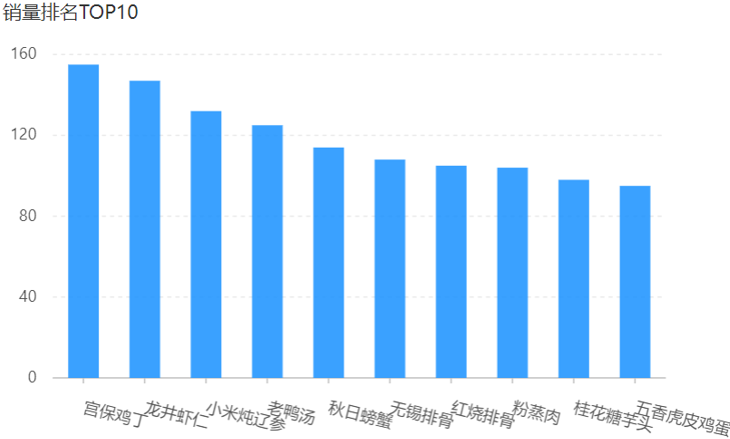
业务功能
- 根据时间选择区间,展示销量前10的商品(包括菜品和套餐)。
- 基于可视化报表的柱状图降序展示商品销量。
- 此处的销量为商品销售的份数。
接口设计
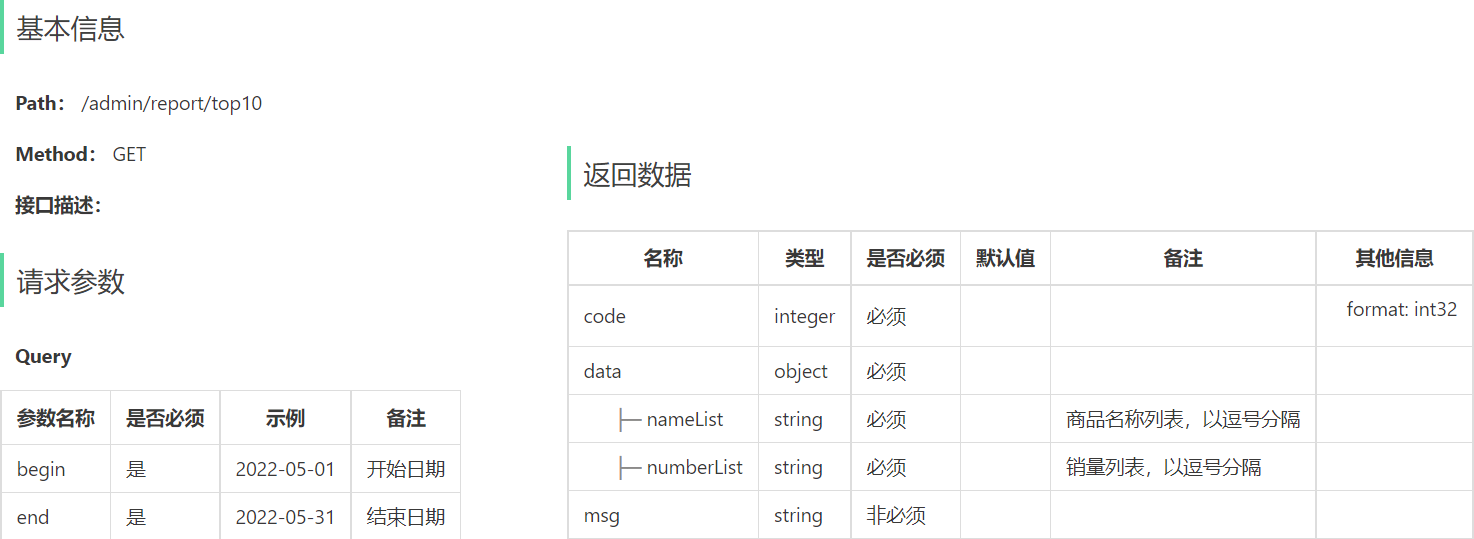
代码开发
- 根据销量排名接口的返回结果设计VO:
@Data
@Builder
@NoArgsConstructor
@AllArgsConstructor
public class SalesTop10ReportVO implements Serializable {
//商品名称列表,以逗号分隔,例如:鱼香肉丝,宫保鸡丁,水煮鱼
private String nameList;
//销量列表,以逗号分隔,例如:260,215,200
private String numberList;
}
- 在ReportController中根据销量排名接口创建top10方法:
@GetMapping("/top10")
@ApiOperation("销量排名top10")
public Result<SalesTop10ReportVO> top10(
@DateTimeFormat(pattern = "yyyy-MM-dd") LocalDate begin,
@DateTimeFormat(pattern = "yyyy-MM-dd") LocalDate end) {
log.info("销量排名top10:{}~{}", begin, end);
SalesTop10ReportVO salesTop10ReportVO = reportService.getSalesTop10(begin, end);
return Result.success(salesTop10ReportVO);
}
- 在ReportService接口中声明getSalesTop10方法:
SalesTop10ReportVO getSalesTop10(LocalDate begin, LocalDate end);
- 根据数据库查询需要设计DTO:
@Data
@AllArgsConstructor
@NoArgsConstructor
@Builder
public class GoodsSalesDTO implements Serializable {
//商品名称
private String name;
//销量
private Integer number;
}
- 在ReportServiceImpl实现类中实现getSalesTop10方法:
@Override
public SalesTop10ReportVO getSalesTop10(LocalDate begin, LocalDate end) {
LocalDateTime beginTime = LocalDateTime.of(begin, LocalTime.MIN); //指定时间范围内最早的时间
LocalDateTime endTime = LocalDateTime.of(end, LocalTime.MAX); //指定时间范围内最晚的时间
List<GoodsSalesDTO> salesTop10 = orderMapper.getSalesTop10(beginTime, endTime); //查询指定时间范围内排名前10名的商品及其销量
List<String> nameList = salesTop10.stream().map(GoodsSalesDTO::getName).collect(Collectors.toList());
List<Integer> numberList = salesTop10.stream().map(GoodsSalesDTO::getNumber).collect(Collectors.toList());
//将集合转换为字符串
String nameListString = StringUtils.join(nameList, ",");
String numberListString = StringUtils.join(numberList, ",");
//构造SalesTop10ReportVO并返回
SalesTop10ReportVO salesTop10ReportVO = SalesTop10ReportVO.builder()
.nameList(nameListString)
.numberList(numberListString)
.build();
return salesTop10ReportVO;
}
- 在OrderMapper接口中声明getSalesTop10方法:
List<GoodsSalesDTO> getSalesTop10(LocalDateTime begin, LocalDateTime end);
- 在OrderMapper.xml文件中编写动态SQL:
<select id="getSalesTop10" resultType="com.sky.dto.GoodsSalesDTO">
select od.name, sum(od.number) number from orders o, order_detail od where od.order_id = o.id and o.status = 5
<if test="begin != null">
and o.order_time > #{begin}
</if>
<if test="end != null">
and o.order_time < #{end}
</if>
group by od.name order by number desc limit 0, 10
</select>
功能测试
可以通过如下方式进行测试:接口文档测试,前后端联调。
标签:第十一天,map,begin,end,String,LocalDate,dateList,外卖,苍穹 From: https://www.cnblogs.com/zgg1h/p/18312998