标签:文件 java 写入 盘点 file new txt out
盘点java写入文件的几种方法
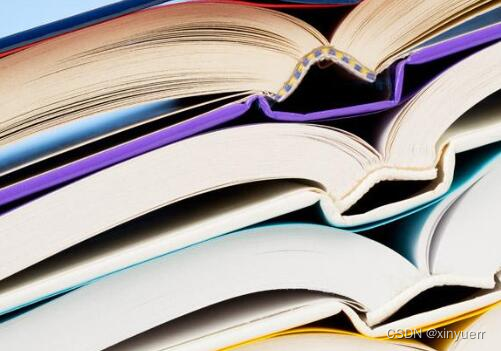
这篇文章主要介绍了java写入文件的几种方法,需要的朋友可以参考下
一,FileWritter写入文件
FileWritter, 字符流写入字符到文件。默认情况下,它会使用新的内容取代所有现有的内容,然而,当指定一个true (布尔)值作为FileWritter构造函数的第二个参数,它会保留现有的内容,并追加新内容在文件的末尾。
1. 替换所有现有的内容与新的内容。
new FileWriter(file);2. 保留现有的内容和附加在该文件的末尾的新内容。
new FileWriter(file,true);
追加文件示例
一个文本文件,命名为“javaio-appendfile.txt”,并包含以下内容。
ABC Hello追加新内容 new FileWriter(file,true)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 | package com.yiibai.file; import java.io.File; import java.io.FileWriter; import java.io.BufferedWriter; import java.io.IOException; public class AppendToFileExample { public static void main( String[] args ) { try { String data = " This content will append to the end of the file" ; File file = new File( "javaio-appendfile.txt" ); //if file doesnt exists, then create it if (!file.exists()){ file.createNewFile(); } //true = append file FileWriter fileWritter = new FileWriter(file.getName(), true ); BufferedWriter bufferWritter = new BufferedWriter(fileWritter); bufferWritter.write(data); bufferWritter.close(); System.out.println( "Done" ); } catch (IOException e){ e.printStackTrace(); } } } |
结果
现在,文本文件“javaio-appendfile.txt”内容更新如下:
ABC Hello This content will append to the end of the file
二,BufferedWriter写入文件
缓冲字符(BufferedWriter )是一个字符流类来处理字符数据。不同于字节流(数据转换成字节),你可以直接写字符串,数组或字符数据保存到文件。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 | package com.yiibai.iofile; import java.io.BufferedWriter; import java.io.File; import java.io.FileWriter; import java.io.IOException; public class WriteToFileExample { public static void main(String[] args) { try { String content = "This is the content to write into file" ; File file = new File( "/users/mkyong/filename.txt" ); // if file doesnt exists, then create it if (!file.exists()) { file.createNewFile(); } FileWriter fw = new FileWriter(file.getAbsoluteFile()); BufferedWriter bw = new BufferedWriter(fw); bw.write(content); bw.close(); System.out.println( "Done" ); } catch (IOException e) { e.printStackTrace(); } } } |
三,FileOutputStream写入文件
文件输出流是一种用于处理原始二进制数据的字节流类。为了将数据写入到文件中,必须将数据转换为字节,并保存到文件。请参阅下面的完整的例子。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 | package com.yiibai.io; import java.io.File; import java.io.FileOutputStream; import java.io.IOException; public class WriteFileExample { public static void main(String[] args) { FileOutputStream fop = null ; File file; String content = "This is the text content" ; try { file = new File( "c:/newfile.txt" ); fop = new FileOutputStream(file); // if file doesnt exists, then create it if (!file.exists()) { file.createNewFile(); } // get the content in bytes byte [] contentInBytes = content.getBytes(); fop.write(contentInBytes); fop.flush(); fop.close(); System.out.println( "Done" ); } catch (IOException e) { e.printStackTrace(); } finally { try { if (fop != null ) { fop.close(); } } catch (IOException e) { e.printStackTrace(); } } } } |
//更新的JDK7例如,使用新的“尝试资源关闭”的方法来轻松处理文件。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 | package com.yiibai.io; import java.io.File; import java.io.FileOutputStream; import java.io.IOException; public class WriteFileExample { public static void main(String[] args) { File file = new File( "c:/newfile.txt" ); String content = "This is the text content" ; try (FileOutputStream fop = new FileOutputStream(file)) { // if file doesn't exists, then create it if (!file.exists()) { file.createNewFile(); } // get the content in bytes byte [] contentInBytes = content.getBytes(); fop.write(contentInBytes); fop.flush(); fop.close(); System.out.println( "Done" ); } catch (IOException e) { e.printStackTrace(); } } } |
下面是其他网友的补充
java.io的几种读写文件的方式
一、java把这些不同来源和目标的数据都统一抽象为数据流。
Java语言的输入输出功能是十分强大而灵活的。
在Java类库中,IO部分的内容是很庞大的,因为它涉及的领域很广泛:标准输入输出,文件的操作,网络上的数据流,字符串流,对象流,zip文件流等等。
这里介绍几种读写文件的方式
二、InputStream、OutputStream(字节流)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | //读取文件(字节流) FileInputStream in = new FileInputStream( "d:\\1.txt" ); //写入相应的文件 FileOutputStream out = new FileOutputStream( "d:\\2.txt" ); //读取数据 //一次性取多少字节 byte [] bytes = new byte [ 2048 ]; //接受读取的内容(n就代表的相关数据,只不过是数字的形式) int n = - 1 ; //循环取出数据 while ((n = in.read(bytes, 0 ,bytes.length)) != - 1 ) { //转换成字符串 String str = new String(bytes, 0 ,n, "UTF-8" ); #这里可以实现字节到字符串的转换,比较实用 System.out.println(str); //写入相关文件 out.write(bytes, 0 , n); //清除缓存向文件写入数据 out.flush(); } //关闭流 in.close(); out.close(); |
三、BufferedInputStream、BufferedOutputStream(缓存字节流)使用方式和字节流差不多,但是效率更高(推荐使用)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | //读取文件(缓存字节流) BufferedInputStream in= new BufferedInputStream( new FileInputStream( "d:\\1.txt" )); //写入相应的文件 BufferedOutputStream out= new BufferedOutputStream( new FileOutputStream( "d:\\2.txt" )); //读取数据 //一次性取多少字节 byte [] bytes = new byte [ 2048 ]; //接受读取的内容(n就代表的相关数据,只不过是数字的形式) int n = - 1 ; //循环取出数据 while ((n = in.read(bytes, 0 ,bytes.length)) != - 1 ) { //转换成字符串 String str = new String(bytes, 0 ,n, "UTF-8" ); System.out.println(str); //写入相关文件 out.write(bytes, 0 , n); //清除缓存,向文件写入数据 out.flush(); } //关闭流 in.close(); out.close(); |
四、InputStreamReader、OutputStreamWriter(字节流,这种方式不建议使用,不能直接字节长度读写)。使用范围用做字符转换
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | //读取文件(字节流) InputStreamReader in = new InputStreamReader( new FileInputStream( "d:\\1.txt" ), "UTF-8" ); //写入相应的文件 OutputStreamWriter out = new OutputStreamWriter( new FileOutputStream( "d:\\2.txt" )); //读取数据 //循环取出数据 char [] chars = new char [ 2048 ]; int len = - 1 ; while ((len = in.read(chars, 0 ,chars.length)) != - 1 ) { System.out.println(len); //写入相关文件 out.write(chars, 0 ,len); //清除缓存 out.flush(); } //关闭流 in.close(); out.close(); |
五、BufferedReader、BufferedWriter(缓存流,提供readLine方法读取一行文本)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | FileInputStream fileInputStream = new FileInputStream( "d:\\1.txt" ); FileOutputStream fileOutputStream = new FileOutputStream( "d:\\2.txt" , true ); InputStreamReader inputStreamReader = new InputStreamReader(fileInputStream, "UTF-8" ); OutputStreamWriter outputStreamWriter = new OutputStreamWriter(fileOutputStream, "UTF-8" ); //读取文件(字符流) BufferedReader in = new BufferedReader(inputStreamReader, "UTF-8" ));#这里主要是涉及中文 //BufferedReader in = new BufferedReader(new FileReader("d:\\1.txt"))); //写入相应的文件 BufferedWriter out = new BufferedWriter(outputStreamWriter, "UTF-8" )); //BufferedWriter out = new BufferedWriter(new FileWriter("d:\\2.txt")); //读取数据 //循环取出数据 String str = null ; while ((str = in.readLine()) != null ) { System.out.println(str); //写入相关文件 out.write(str); out.newLine(); //清除缓存向文件写入数据 out.flush(); } //关闭流 in.close(); out.close(); |
六、Reader、PrintWriter(PrintWriter这个很好用,在写数据的同事可以格式化)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | //读取文件(字节流) Reader in = new InputStreamReader( new FileInputStream( "d:\\1.txt" ), "UTF-8" ); //写入相应的文件 PrintWriter out = new PrintWriter( new FileWriter( "d:\\2.txt" )); //读取数据 //循环取出数据 byte [] bytes = new byte [ 1024 ]; int len = - 1 ; while ((len = in.read()) != - 1 ) { System.out.println(len); //写入相关文件 out.write(len); //清除缓存 out.flush(); } //关闭流 in.close(); out.close(); |
七、基本的几种用法就这么多,当然每一个读写的使用都是可以分开的。为了更好的来使用io。流里面的读写,建议使用BufferedInputStream、BufferedOutputStream
转自:微点阅读 https://www.weidianyuedu.com
原文链接:https://blog.csdn.net/xinyuerr/article/details/132870698
标签:文件,
java,
写入,
盘点,
file,
new,
txt,
out
From: https://www.cnblogs.com/sunny3158/p/17914473.html