https://www.geeksforgeeks.org/arrays-sort-in-java-with-examples/
Array class is a class containing static methods that are used with arrays in order to search, sort, compare, insert elements, or return a string representation of an array. So let us specify the functions first and later onwards we will be discussing the same. They are as follows being present in java.util.Arrays class. Here we will be discussing different plots using the sort() method of the Arrays class.
Arrays.sort() method consists of two variations one in which we do not pass any arguments where it sort down the complete array be it integer array or character array but if we are supposed to sort a specific part using this method of Arrays class then we overload it and pass the starting and last index to the array.
Syntax: sort() Method
Arrays.sort();
Syntax: Overloaded sort() Method
<iframe data-google-container-id="2" data-is-safeframe="true" data-load-complete="true" frameborder="0" height="1" id="google_ads_iframe_/27823234/GFG_Desktop_PostContent_336x280_0" marginheight="0" marginwidth="0" scrolling="no" src="https://8eeb73d3905dad9c6c0af9af8b9d7f66.safeframe.googlesyndication.com/safeframe/1-0-40/html/container.html" title="3rd party ad content" width="1"></iframe>public static void sort(int[] arr, int from_Index, int to_Index) ;
Parameters: It takes three parameters as can be perceived from the syntax which is as follows:
- The array to be sorted
- The index of the first element, inclusive, to be sorted (Referred to as from_index)
- The index of the last element, exclusive, to be sorted (Referred to as last_index)
Return Type: NA
Complexity Analysis:
- Time Complexity: O(N log N)
- Auxiliary Space: O(1)
Now let us see the implementation of the sort() function across different scenarios of the Arrays class as follows:
Example 1:
- Java
import java.util.Arrays;
class GFG {
public static void main(String args[])
{
int [] arr = { 5 , - 2 , 23 , 7 , 87 , - 42 , 509 };
System.out.println( "The original array is: " );
for ( int num : arr) {
System.out.print(num + " " );
}
Arrays.sort(arr);
System.out.println( "\nThe sorted array is: " );
for ( int num : arr) {
System.out.print(num + " " );
}
}
}
|
The original array is: 5 -2 23 7 87 -42 509 The sorted array is: -42 -2 5 7 23 87 509
Time Complexity: O(nlog(n)) as it complexity of arrays.sort()
Auxilliary Space : O(1)
Example 2:
- Java
// Java Program to Sort Array of Integers
// by Default Sorts in an Ascending Order
// using Arrays.sort() Method
// Importing Arrays class from the utility class
import java.util.Arrays;
// Main class
public class GFG {
// Main driver method
public static void main(String[] args)
{
// Custom input array
int [] arr = { 13 , 7 , 6 , 45 , 21 , 9 , 101 , 102 };
// Applying sort() method over to above array
// by passing the array as an argument
Arrays.sort(arr);
// Printing the array after sorting
System.out.println( "Modified arr[] : "
+ Arrays.toString(arr));
}
}
|
Modified arr[] : [6, 7, 9, 13, 21, 45, 101, 102]
Time Complexity: O(N log N)
Auxiliary Space: O(1)
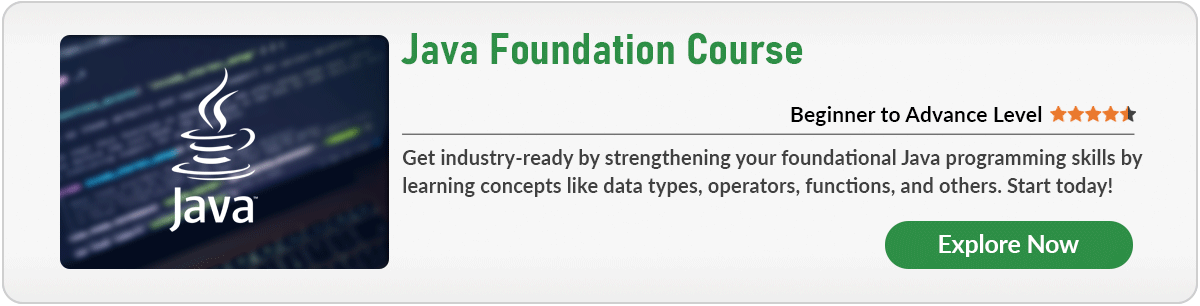
Example 3:
- Java
// Java program to Sort a Subarray in Array
// Using Arrays.sort() method
// Importing Arrays class from java.util package
import java.util.Arrays;
// Main class
public class GFG {
// Main driver method
public static void main(String[] args)
{
// Custom input array
// It contains 8 elements as follows
int [] arr = { 13 , 7 , 6 , 45 , 21 , 9 , 2 , 100 };
// Sort subarray from index 1 to 4, i.e.,
// only sort subarray {7, 6, 45, 21} and
// keep other elements as it is.
Arrays.sort(arr, 1 , 5 );
// Printing the updated array which is
// sorted after 2 index inclusive till 5th index
System.out.println( "Modified arr[] : "
+ Arrays.toString(arr));
}
}
|
Modified arr[] : [13, 6, 7, 21, 45, 9, 2, 100]
Time Complexity: O(nlog(n)) as it complexity of arrays.sort()
Auxilliary Space : O(1)
Example 4:
- Java
// Java program to Sort a Subarray in Descending order
// Using Arrays.sort()
// Importing Collections class and arrays classes
// from java.util package
import java.util.Arrays;
import java.util.Collections;
// Main class
public class GFG {
// Main driver method
public static void main(String[] args)
{
// Note that we have Integer here instead of
// int[] as Collections.reverseOrder doesn't
// work for primitive types.
Integer[] arr = { 13 , 7 , 6 , 45 , 21 , 9 , 2 , 100 };
// Sorts arr[] in descending order using
// reverseOrder() method of Collections class
// in Array.sort() as an argument to it
Arrays.sort(arr, Collections.reverseOrder());
// Printing the array as generated above
System.out.println( "Modified arr[] : "
+ Arrays.toString(arr));
}
}
|
Modified arr[] : [100, 45, 21, 13, 9, 7, 6, 2]
Time Complexity: O(nlog(n)) as it complexity of arrays.sort()
Auxilliary Space : O(1)
Example 5:
- Java
// Java program to sort an array of strings
// in ascending and descending alphabetical order
// Using Arrays.sort()
// Importing arrays and Collections class
// from java.util class
import java.util.Arrays;
import java.util.Collections;
// Main class
public class GFG {
// Main driver method
public static void main(String[] args)
{
// Custom input string
String arr[] = { "practice.geeksforgeeks.org" ,
"www.geeksforgeeks.org" ,
"code.geeksforgeeks.org" };
// Sorts arr[] in ascending order
Arrays.sort(arr);
System.out.println( "Modified arr[] : "
+ Arrays.toString(arr));
// Sorts arr[] in descending order
Arrays.sort(arr, Collections.reverseOrder());
// Lastly printing the above array
System.out.println( "Modified arr[] :"
+ Arrays.toString(arr));
}
}
|
Modified arr[] : Modified arr[] :[www.geeksforgeeks.org, practice.geeksforgeeks.org, code.geeksforgeeks.org]
Time Complexity: O(nlog(n)) as it complexity of arrays.sort()
Auxilliary Space : O(1)
Now lastly we will be implementing the sort() method to the fullest because here we will be declaring our own defined criteria with the help of the Comparator interface.
Example 6:
- Java
// Java program to demonstrate Working of
// Comparator interface
// Importing required classes
import java.io.*;
import java.lang.*;
import java.util.*;
// Class 1
// A class to represent a student.
class Student {
int rollno;
String name, address;
// Constructor
public Student( int rollno, String name, String address)
{
// This keyword refers to current object itself
this .rollno = rollno;
this .name = name;
this .address = address;
}
// Used to print student details in main()
public String toString()
{
return this .rollno + " " + this .name + " "
+ this .address;
}
}
// Class 2
// Helper class extending Comparator interface
class Sortbyroll implements Comparator<Student> {
// Used for sorting in ascending order of
// roll number
public int compare(Student a, Student b)
{
return a.rollno - b.rollno;
}
}
// Class 3
// Main class
class GFG {
// Main driver method
public static void main(String[] args)
{
Student[] arr
= { new Student( 111 , "bbbb" , "london" ),
new Student( 131 , "aaaa" , "nyc" ),
new Student( 121 , "cccc" , "jaipur" ) };
System.out.println( "Unsorted" );
for ( int i = 0 ; i < arr.length; i++)
System.out.println(arr[i]);
// Sorting on basic as per class 1 created
// (user-defined)
Arrays.sort(arr, new Sortbyroll());
System.out.println( "\nSorted by rollno" );
for ( int i = 0 ; i < arr.length; i++)
System.out.println(arr[i]);
}
}
|
Unsorted 111 bbbb london 131 aaaa nyc 121 cccc jaipur Sorted by rollno 111 bbbb london 121 cccc jaipur 131 aaaa nyc
Time Complexity: O(nlog(n)) as it complexity of arrays.sort()
Auxilliary Space : O(1)
Remember: There is a slight difference between Arrays.sort() vs Collections.sort(). Arrays.sort() works for arrays which can be of primitive data type also. Collections.sort() works for objects Collections like ArrayList, LinkedList, etc.
Using the reverse order method: This method will sort the array in the descending. In Java Collections class also provides the reverseOrder() method to sort the array in reverse-lexicographic order. It does not parse any parameter because static method, so we can invoke it directly by using the class name. it will sort arrays in the ascending order by the sort() method after that the reverse order() method will give us the natural ordering and we will get the sorted array in the descending order.
Syntax:
Arrays.sort(a, Collections.reverseOrder());
Example 7:
- Java
// This will sort the array in the descending order
/*package whatever //do not write package name here */
import java.util.Arrays;
import java.util.Collections;
public class GFG {
public static void main(String[] args)
{
Integer[] array
= { 99 , 12 , - 8 , 12 , 34 , 110 , 0 , 121 , 66 , - 110 };
Arrays.sort(array, Collections.reverseOrder());
System.out.println(
"Array in descending order: "
+ Arrays.toString(array));
}
}
|
Array in descending order: [121, 110, 99, 66, 34, 12, 12, 0, -8, -110]
Time Complexity: O(nlog(n)) as it complexity of arrays.sort()
Auxilliary Space : O(1)
This article is contributed by Mohit Gupta. If you like GeeksforGeeks and would like to contribute, you can also write an article using write.geeksforgeeks.org or mail your article to review-team@geeksforgeeks.org. See your article appearing on the GeeksforGeeks main page and help other Geeks.
标签:sort,arr,Java,Arrays,method,array,class From: https://www.cnblogs.com/kungfupanda/p/17018068.html